This Java based development framework makes developing a (enterprise) web app as easy as defining your data model. Your main task is to create a good data model, pour it into classes, and defining the relationships and referential integrity. The framework creates the web app for you! When you are ready to deploy, the web app can be hosted in a Tomcat environment.
Gliffy diagram & flowcharting
I am using Gliffy a lot. It is a great graphic tool (free Chrome extension) to create flowcharts, UML diagrams, network diagrams and much more. Very important is the rubber-banding support: when you connect objects using their red indicated connections (during drawing), these connections are maintained wherever you drag the objects.
https://chrome.google.com/webstore/detail/gliffy-diagrams/bhmicilclplefnflapjmnngmkkkkpfad
Node.js: Application as a Windows service
node-windows
node-windows is a standalone module that makes it possible to offer a Node.js script as native Windows services..
Prerequisite: You have succesfully installed Node.js.
Install with npm using the global flag:
npm install -g node-windows
In the project root run:
npm link node-windows
Hello world example
Create a hello.js file with this code (Hello World sample from the Express website):
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => res.send('Hello World!'))
app.listen(port, () => console.log(`Example app listening at http://localhost:${port}`))
You can (should) test if it works by running it from the command line:
node hello.js
If there are no errors, point your browser to http://localhost:3000. If it says Hello World! you are well on your way!
Script to create the service
Now we have our app, we want to make it a service. This is achieved by another script we call hello-windows-service.js:
var Service = require('node-windows').Service;
// Create a new service object
var svc = new Service({
name:'Node app hello',
description: 'Node app hello as Windows Service',
script: 'C:\\npm\\.node_modules_global\\hello.js'
});
// Listen for the "install" event, which indicates the
// process is available as a service.
svc.on('install',function(){
svc.start();
});
svc.install();
Most important is the correct location of the script in the Service call..
Now run this script to install the service into Windows:
node hello-windows-service.js
Now if you check your services, I hope you’ll find this:

Great! The Node app is running as a Windows service under a local system account. The app is running even when nobody is logged in. Goal achieved. Oh and because it’s on Automatic start, it wil always become available after each server restart.
Based on this article by Peter Eysermans.
Node.js: Install
Node.js org offers a complete install package at https://nodejs.org/en/download/ .
The install is a complete developer’s package that sets you up with a complete set of tools you need to develop and build Node.js apps.
The install package includes:
The package is delivered through a Chocolatey package, and is completely unattended (so well written installer!).
m1m0 learn to code
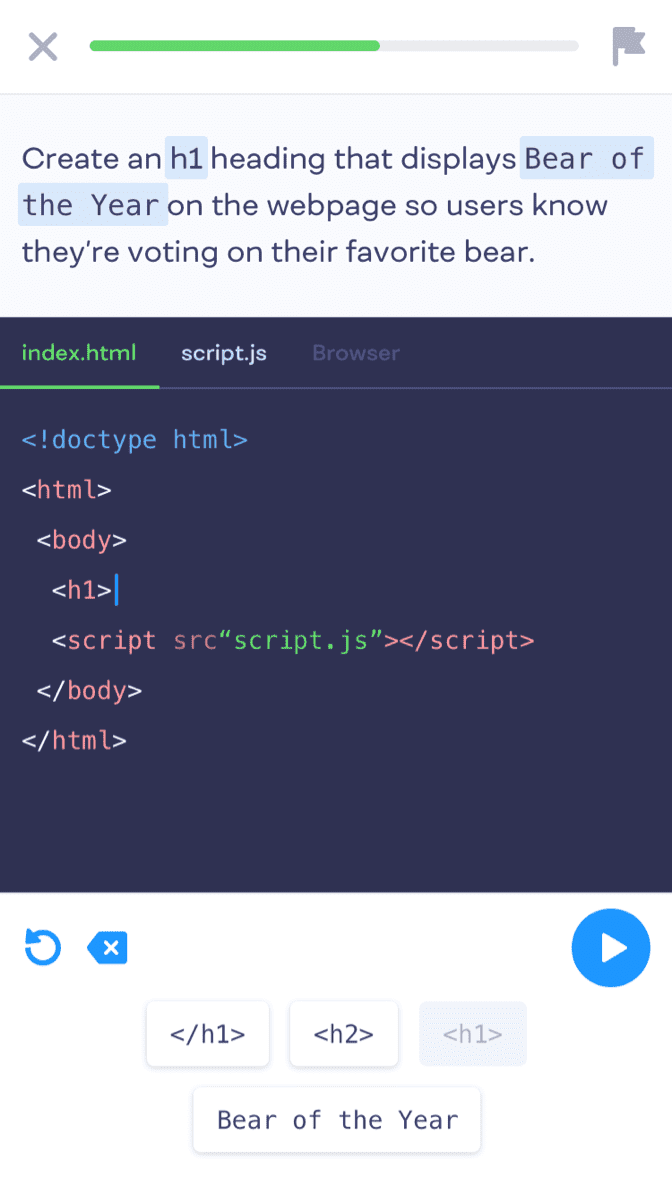
An article in Computer Totaal mentioned this App. It is said to be a kind of game to learn programming bit by bit. By solving puzzles you gain points, thus rewarded for learning. The App gives you languages like HTML, CSS, SQL, Java, Python, Ruby, C++ and more. I haven’t tried it yet, but it looks like fun.
React: build for relative paths
If it’s not a real production build, you probably test your React Apps in a sub folder at your hosting space. The information on this is scattered and explained way too difficult. There’s nothing to it:
- Open package.json in uour App root folder
- Find the parameter “homepage” or add it (image above)
- Give the parameter the value of the complete path where your app will be hosted (image above)
- Save the package.json file
- Build the React app using npm start build
- Upload the production build (folder build) to your hosting provider
- And done!
And when you are building the React App using npm run build, it should confirm the subfolder:
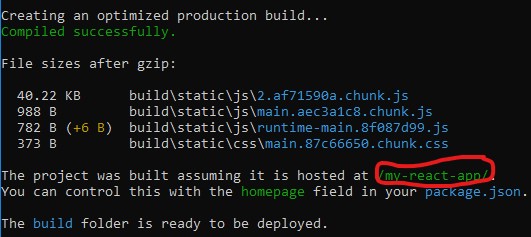
FTP: FileZilla
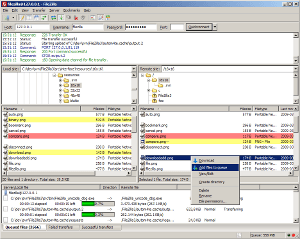
The free FTP solution
FileZilla has been around for quite a while now. It’s an unbeatable combination: it does what it should, and it’s free!
Thanks sponsors! I’ve visited your pages 😉
Outgoing port tester: PortQuiz.net
I needed to test whether some ports were enabled or blocked. This tool/ service is great: It simply accepts any port request and tells you if it succeeded. From the PortQuiz page:
This server listens on all TCP ports, allowing you to test any outbound TCP port.
You have reached this page on port 80.
Your network allows you to use this port. (Assuming that your network is not doing advanced traffic filtering.)
Network service: http
Your outgoing IP: 86.93.90.146
Test a port using a command
$ telnet portquiz.net 80 Trying ... Connected to portquiz.net. Escape character is '^]'.
$ nc -v portquiz.net 80 Connection to portquiz.net 80 port [tcp/daytime] succeeded!
$ curl portquiz.net:80 Port 80 test successful! Your IP: 86.93.90.146
$ wget -qO- portquiz.net:80 Port 80 test successful! Your IP: 86.93.90.146
# For Windows PowerShell users PS C:\> Test-NetConnection -InformationLevel detailed -ComputerName portquiz.net -Port 80
Test a port using your browser
In your browser address bar:Â http://portquiz.net:XXXX
Examples:
http://portquiz.net:8080
http://portquiz.net:8
http://portquiz.net:666
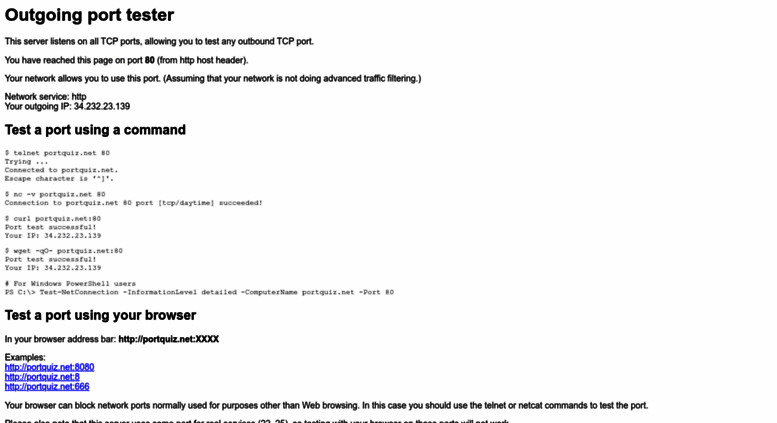
Clipboard enhancement
You can use the new Windows 10 clipboard tool with the combination Windows-Shift-S (Try it now). It has nice possibilities like selecting a rectangle, free shape, current window or complete screen. But I want more. For instance: a form of history than can easily be viewed and selected. I found this nice software that can even clip and paste cross platform (haven’t tested it yet) through Google cloud storage.
Enter the free 1Clipboard. You clip whatever you want using Windows-Shift-S. After that, you can see your last clip in the Windows message center. With the key combination Ctrl-Alt-V the program opens, showing you the clipboard history and allowing you to select any clip (which is put onto the clipboard when you click it), or make it a favorite. There is even a search available.
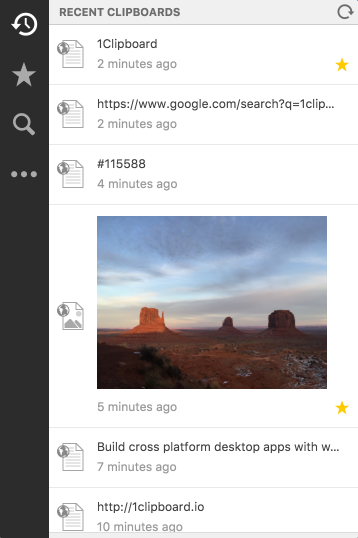
Remote control software
I am using Teamviewer professionally, but It’s pricey and not very well put together. We have a branded quicksupport online, so I receive an e-mail whenever someone starts this program. The e-mail contains a link to the handling system, that is online and you need to log-in to it. There I can open the request which at its turn opens Teamviewer on my desktop. Sometimes the connection will be made instantly without problems, only asking the service requester whether I’m allowed to take over. But lots of times, there are hiccups during the Process and it breaks. We have to try over again way too much times. Furthermore, when it works, I have helped and ended the session even filled the actions taken, the ticket is not closed!? Even worse: the ticket is still in the handling queue waiting to be assigned and treated. Silly software. Who programs this sh*t?
Anyway, I was looking for free software to take over the console as we have some special services computers that need to stay logged-in in the console mode, and it seems that Windows 10 has dropped support for the RDP (mstsc) console access. The program I have tried somewhat successful (1 night testing):
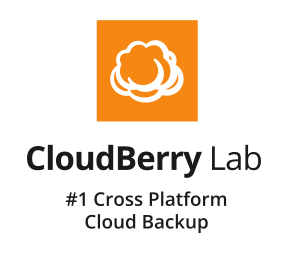
MSP360â„¢ Cloudberry Remote Assistance
This software has the following features:
- It uses a central server, so remote support can be initiated anywhere (internet needed)
- It does not need any port forwarding or firewall exclusions
- It is free software
- It allows remote control without user interaction, so you can take over unattended desktops
- There is a rather new iOS client (that does not work at this time)
Link to the MSP360 page.